In today's fast-paced business environment, streamlining processes and workflows is key to staying ahead of the competition. One such process that can be streamlined is the creation of service tickets.
ServiceNow, a leading cloud-based platform for IT service management, provides a powerful API that allows developers to automate and simplify the process of ticket creation. So, whether you're an IT professional or a developer looking to optimize your workflow, read on to discover how the ServiceNow API can help you create tickets faster and take your service management to the next level.
Setup
To make use of the API, you first need an API account in ServiceNow. If you want to create your own Developer instance for testing, it is free to do so and can be done here. For the further demonstrations, we are using default configurations that can be found in any Developer instance of ServiceNow. To create an API account in ServiceNow, you can follow the below steps:
1. Login to your ServiceNow Instance and Go to the Users section and click “New” in the top Right.
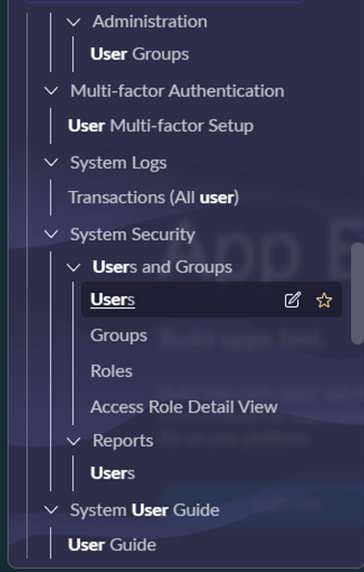
2. Input a user ID and optional first name for your API account, then check the “Web service access only” checkbox. Once done, click submit.
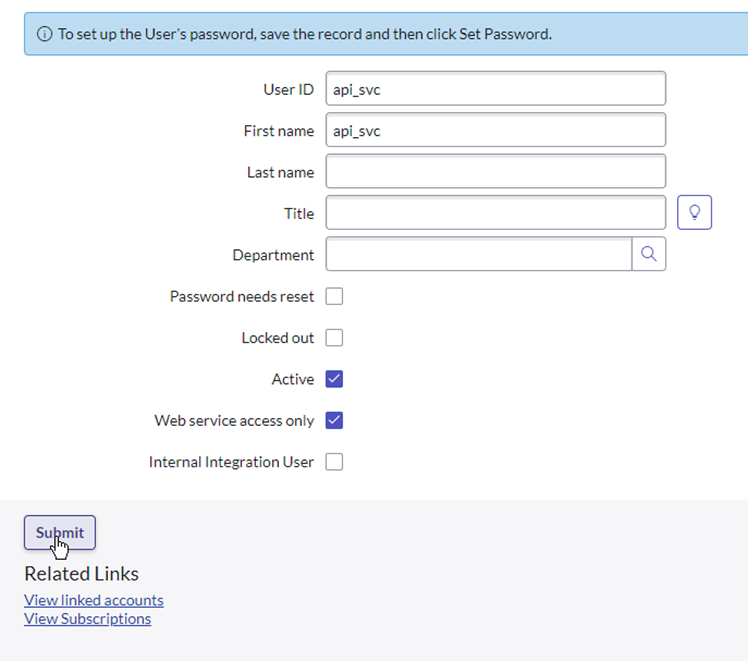
3. Once created, find the account in the users list, and click on it to manage it. Afterwards, in the top right, click “Set Password.”
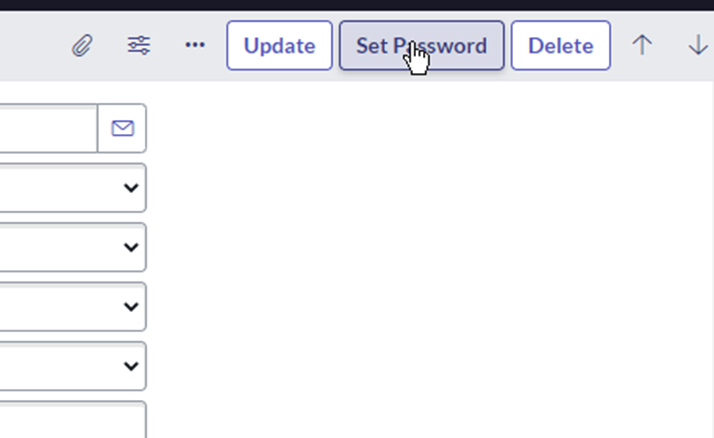
4. In the prompt, click “Generate”, copy the generated password, then click “Save Password.”
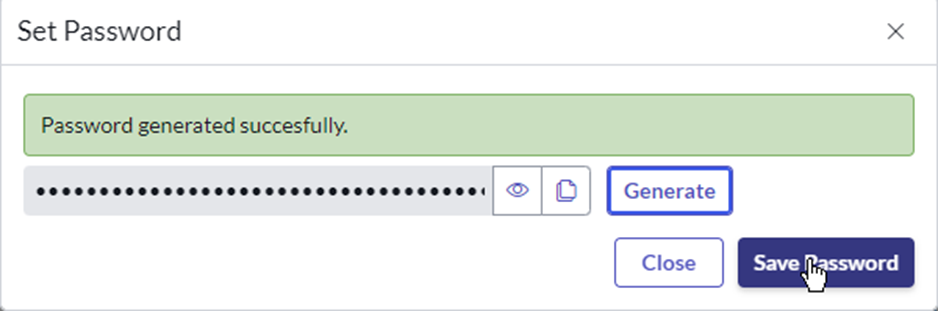
5. Now, add the roles desired by clicking “Edit…” in the roles tab towards the bottom of the user’s configuration. For testing purposes, we just use the admin, rest_api_explorer, and web_service_admin roles. Below is a brief explanation of each role:
admin: Grants special access to all system functions, data, and features.
web_service_admin: Able to read, create, modify, and delete web service resources.
rest_api_explorer: Grants access to the API
It is important to note that providing these roles to a service account is not a best practice. However, the account does require the rest_api_explorer to use the API.

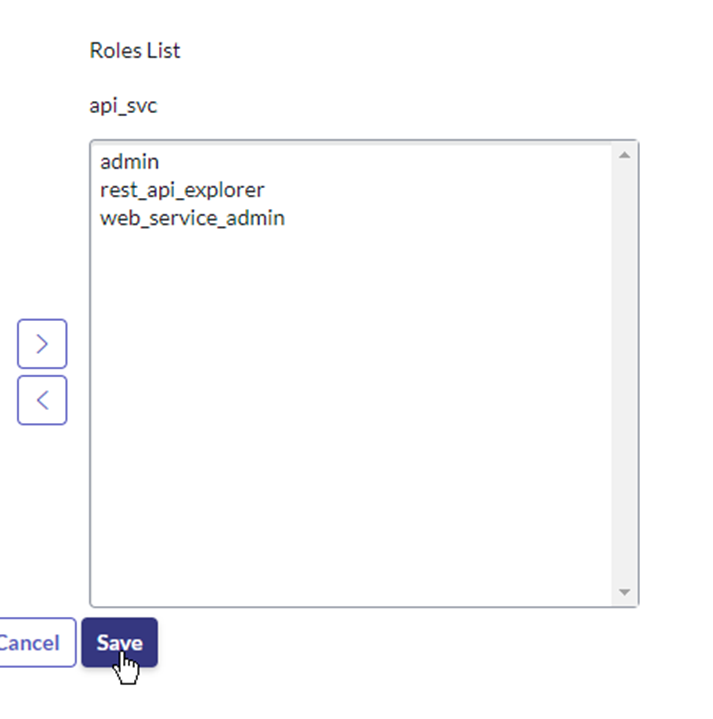
Once the API account is set up and has the proper permission to create the tickets that are required for your use case, you are ready to start making your, or your end users, lives easier! We'll now take a look at how to make requests to the API, what the data looks like, and some things to look out for when using the API. All the code used in the following examples are written in Python but can easily be converted to a different programming language that suits you as the API endpoints work the same regardless. The code for this blog can be found here.
API Authentication
The first step to using the ServiceNow API is to make sure you can properly authenticate. The ServiceNow API can use three types of authentication, including, basic, mutual, and OAuth. Ordered in least secure, to most secure. For our purposes, and for simplicity, we will just cover basic authentication. To authenticate to the API using basic authentication, all you need is the username a password of the account that will be used for API access. Authentication is used in all API requests as shown in the example below. The auth parameter is where the username and password are being used for the basic authentication.
requests.get(url, auth=(username, password), headers=headers)
Generating Tickets With the API
Now that we have a handle on authentication with the API, we can move on to the fun stuff, creating the tickets! Creating tickets in your environment may be a little different from what is being shown depending on how the environment is configured. Each type of ticket will usually have their own API endpoint. However, there may be an instance where you just have to use the generic ‘table’ endpoint, like when creating incidents. Below you will find examples of how to create a ticket for incidents, change requests, and service catalog requests. If you are unfamiliar with making API calls with Python or a different language, you can always use tools like Postman. It will do most of the heavy lifting for you. ServiceNow does have great documentation around their API if you are looking for something specific, which can be found here.
Incident Requests
The example below outlines how to create an incident. This process uses the table API. If the request is successfully created, it will print the response to the screen.
payload = {
'short_description': 'This is a short description',
'summary': 'This is a summary'
} # Define the payload for creating the incident
table_name = 'incident' # Define the table name that will be modified
try:
url = f'{base_url}/api/now/table/{table_name}' # Define the URL for the request
response = requests.post(url, auth=(username, password), headers=headers, data=json.dumps(payload),
verify=verify) # Add the item to the ServiceNow table
if response.status_code == 201: # If the item was successfully added to the table
print(json.dumps(response.json(), indent=4))
else:
print(f'There was a problem adding an entry to the ServiceNow table "{table_name}". '
f'{response.text} : {response.status_code}')
except Exception as e:
print(e)
Change Requests
The example below outlines how to create a normal change request. As with incidents, if the request is successfully created, it will print the response to the screen.
payload = {
'short_description': 'This is a short description',
'summary': 'This is a summary'
} # Define the payload for creating the change request
try:
url = f'{base_url}/api/sn_chg_rest/change/normal' # Define the URL for the request
response = requests.post(url, auth=(username, password), headers=headers, data=json.dumps(payload),
verify=verify) # Create the ServiceNow Change Request
if response.status_code == 200: # If the change was successfully created
print(json.dumps(response.json(), indent=4))
else:
print(f'There was a problem creating the ServiceNow Change Request. '
f'{response.text} : {response.status_code}')
except Exception as e:
print(e)
Service Catalog Requests
Lastly, we have the service catalog requests. This process will first get a list of all service catalog items and check if the one we want to create was in the list based on the name of the item. If it was, we use the sys_id of the item to create the request.
payload = {
'sysparm_quantity': 1,
'variables': {
}
} # Define the payload for creating the request
sc_item_name = 'Standard Laptop' # Define the Service Catalog item name
try:
url = f'{base_url}/api/sn_sc/servicecatalog/items' # Add them to the URL
response = requests.get(url, auth=(username, password), headers=headers,
verify=verify) # Get the Service Catalog item(s)
if response.status_code == 200: # If the data was successfully retrieved
items = {x['name']: x['sys_id'] for x in response.json()['result']} # Create dictionary mapping of service catalog item to sys_id
if sc_item_name in items.keys(): # If the item was found
sc_req_item_id = items[sc_item_name] # Get the system ID of the item
url = f'{base_url}/api/sn_sc/servicecatalog/items/{sc_req_item_id}/order_now' # Define the URL for the request
response = requests.post(url, auth=(username, password), headers=headers, data=json.dumps(payload),
verify=verify) # Create the request
if response.status_code == 200: # If the item was successfully created
print(json.dumps(response.json(), indent=4))
else:
print(f'There was a problem creating the ServiceNow Request. '
f'{response.text} : {response.status_code}')
else:
print(f'There was a problem retrieving the ServiceNow catalog items. '
f'{response.text} : {response.status_code}')
except Exception as e:
print(e)
Conclusion
In conclusion, leveraging the ServiceNow API to create tickets is a powerful tool that can help streamline your service management processes and improve overall efficiency. By automating ticket creation, you can reduce the time and effort required to manage service requests. Allowing you, your team, or whoever else may be creating tickets, get valuable time back in their day. We hope that the information provided in this article can help you begin using the ServiceNow API to create tickets. So, what are you waiting for? Start exploring the possibilities of the ServiceNow API and revolutionize the way you manage service requests!
Thank you for taking the time to review this article. Feel free to contact us if your project needs more advanced capabilities.